Do you know how computers perform calculations on numbers? Perhaps you've heard of the binary system and know that it’s the system computers use for storing numbers, and that they can perform any operation upon them. We’re not going to explore the intricacies of positional numeral systems here, but we will say that the numbers handled by modern computers are of two types:
- integers, that is, those which are devoid of the fractional part;
- floating-point numbers (or simply floats), that contain (or are able to contain) the fractional part.
This definition is not entirely accurate but good enough for our purposes. This distinction is very important and the boundary between these two types of numbers is very strict. Both of these kinds of numbers significantly differ in how they are stored in a computer’s memory and in the range of acceptable values. Furthermore, the characteristic of a number which determines its kind, range and application is called a type.
At this point we have made friends with two types of the “C” language – an integer type (known as int) and a floating point type (known as float).
For now, let's leave the floating-point numbers aside (we’ll get back to them soon) and let’s consider the question, maybe a bit banal at first glance, of how the “C” language recognizes integers.
Well, it’s almost the same way that you're used to writing them with a pencil on paper – it’s simply a string of digits that make up the number. But there’s a reservation – you must not insert any characters that are not digits inside the number. Take for example the number eleven million one hundred and eleven thousand one hundred and eleven. If you took a pencil in your hand right now, you would write the number like this:
11,111,111
or (if you are a Pole or a German) like this:
11.111.111
or (if you are a Pole or a German) like this:
11 111 111
Obviously, this makes it easier to read if the number is made up of many digits. However, in “C” it’s prohibited. You must write this number as follows:
11111111
Otherwise you'd expose yourself to some biting remarks from the compiler. How do we code negative numbers in “C”? As usual – by adding a minus. You can write:
-11111111
Positive numbers don't need to be preceded by the plus sign but you can do it if you want. The following lines describe the same number:
+123
123
For now, we’ll deal only with integers – we’ll introduce floating-point numbers in the next chapter.
There are two additional conventions, unknown to the world of mathematics. The first one allows us to use the numbers in an octal representation. If an integer number is preceded by the 0 digit, it will be treated as an octal value. This means that the number must contain digits taken from the [0..7] range only.
0123
is an octal number with a decimal value equal to 83.
0x123
is a hexadecimal number with a decimal value equal to 291.
Maybe you want to see the result of your computation. We’ll discuss this later, but now's a good moment to mention how to print the value of a number.
Well, to print an integer number, you should use (this is only a simple form):
printf("%d\n", IntegerNumberOrExpression);
To print a floating point number, you should use (this is only a simple form):
printf("%f\n", FloatNumberOrExpression);
In both cases, you should first include the stdio header file (as we did in the first program):
#include
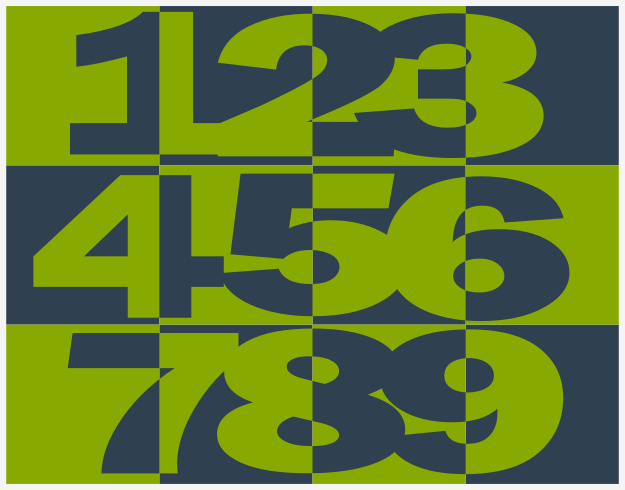
0 Comments
Tell us your queries or more topics which you want